Fixing Locking and Blocking Issues in SQL Server - Part 6 - Avoiding Deadlocks
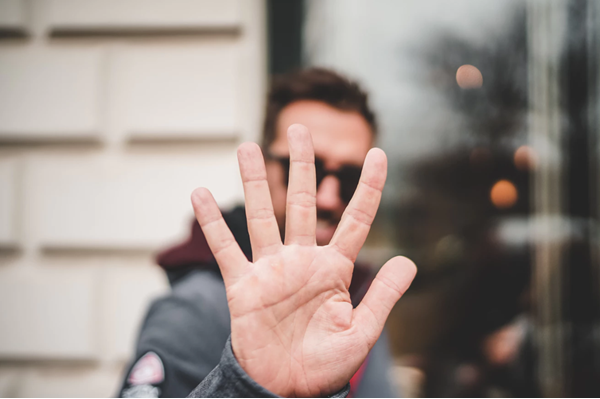
This is part 6 in a series of posts:
- Part 1 covered being sure there is a locking and blocking issue
- Part 2 covered the impact of RCSI
- Part 3 looked at the impacts of indexing on locking and blocking
- Part 4 looked at what deadlocks really are and how SQL Server handles them
- Part 5 looked at how applications should handle deadlocks
Today, though, I want to look at how to try to avoid deadlocks in the first place.
You Can’t
Let’s start with the bad news. You can’t totally avoid deadlocks without doing something like serializing all access to the database.
As I mentioned in Part 5, you need to expect and handle deadlocks when they occur.
Now we have the bad news out of the way, let’s look at things that can help to minimize them. Even though we make our applications capable of handling them, I want to see as few of them happening as possible.
Fix the query performance
Part one of this series talked a bit about this. I often see significant blocking issues (and associated deadlocks) completely disappear when the queries run quickly.
The longer that a query runs for, the longer it’s likely to hold locks. We want to minimize that time. Indexing is your friend here.
Minimize the locking (volume and duration)
The fewer things that are blocked, and the shorter time that they’re blocked, the less chance there are for deadlocks in the first place. There are four key aspects of this:
First, (no surprise), indexing is a key issue here. If you don’t give SQL Server suitable indexes to let it find just the data that you need to lock, you shouldn’t be surprised when it ends up locking entire partitions or tables.
Second, the design of your tables is also an issue. A properly normalized database schema is easier to work with in terms of minimizing locks.
Third, you need to use the lowest isolation level that works for your queries. Part two of this series talked about using RCSI. Often that will really help. Your biggest pain point though is going to come from applications that insist on using Serializable as an isolation level. Unfortunately, many Microsoft applications default to using this (I’m looking at you Biztalk’s SQL Adapter), as do many other things in Windows like Component Services. Whenever you have a choice and it’s appropriate, reduce the isolation level to the lowest you can work with.
You might be wondering about the use of NOLOCK here. Clearly it might help if you can deal with the risk. Basically, you’re giving up consistency for reduced locking and performance. If you’re working only with archive data that isn’t changing, it might be an ok option.
If you’re working with data that’s being actively changed, it’s a significant risk. It’s hard to explain duplicated or missing rows on reports, and even harder to explain data on your report that doesn’t exist in the database because it was rolled back.
I also see people trying to put NOLOCK on INSERT, UPDATE, DELETE. Here’s a hint: that’s not going to work.
Fourth, you need to hold locks for the shortest time that you possibly can. I’m not talking about holding locks across user interactions, that would be just plain dumb. You need to get as much work done as quickly as possible. If you want high concurrency, holding transactions across multiple round trips to the database server isn’t clever, yet I see it all the time.
Don’t create a proc to insert an order header, then another proc to insert an order detail line, and then call them one by one within a transaction started by the client application. Instead, pass the whole order (or even multiple orders where it makes sense) directly to a procedure on the SQL Server that does the whole job at once. Table-valued parameters are your friend here.
Locks are typically happening within transactions so you need to keep the transaction durations very short. And that means that you need to manage them properly. Start them only when needed and commit them or roll them back as soon as possible.
The transaction handling in JDBC is a great example of how not to handle transactions.
It turns on chained mode (which means that SQL Server starts a transaction automagically when a data modification occurs), and then just periodically runs a command that says “if there is a transaction, commit it”. Please don’t do this. At least not if you’re looking for high performance and/or concurrency.
Serialize the workload
One useful, (but possibly contentious) method of reducing deadlocks, is to try to ensure that you lock objects in the database in the same order within all your logic.
I remember a site that I went to in the 1980s where they had a rule that tables were always used in alphabetical order. That sounded like a bad idea at the time, and it still sounds like a bad idea.
However, you can make a big difference to deadlocks by using tables in the same logical order every time. For example, if you acquire an order number from a sequence, then using it in an order header, then write the order detail lines, write an audit entry, etc. and keep to that same type of order, you’ll have a lot less deadlocks to deal with, as it will naturally serialize the work to be done.
When all else fails
Even with all the above, you still will likely have some deadlocks. Apart from all the handling options, you might consider exerting some control over who loses when a deadlock occurs. Perhaps you have a background task that you don’t mind dying and restarting. In that case, you can set its deadlock priority low so it offers itself as a victim, rather than just having SQL Server work out who’s written the least to the transaction log.
In the next post, I’ll look at retry logic at the server end.
2019-05-23